http://stackoverflow.com/questions/13190137/location-detection-in-web-browser https://www.w3schools.com/html/html5_geolocation.asp
The Geolocation API provides a method to locate the user’s exact (more or less – see below) position. This is useful in a number of ways ranging from providing a user with location specific information to providing route navigation.
Although it’s not actually part of the HTML5 specification, as it was developed as a separate specification by the W3C, rather than the WHATWG, if the esteemed HTML5 Doctors Remy Sharp and Bruce Lawson felt it was fitting enough to include in their book, then it’s perfectly ok to write about it here.
The API is actually remarkably simple to use and this article aims to introduce the API and show just how easy it is.
Browser Compatibility
Currently the W3C Geolocation API is supported by the following desktop browsers:
- Firefox 3.5+
- Chrome 5.0+
- Safari 5.0+
- Opera 10.60+
- Internet Explorer 9.0+
There is also support for the W3C Geolocation API on mobile devices:
- Android 2.0+
- iPhone 3.0+
- Opera Mobile 10.1+
- Symbian (S60 3rd & 5th generation)
- Blackberry OS 6
- Maemo
Data Protection
The specification explicitly states that since the nature of the API also exposes the user’s location and therefore could compromise their privacy, the user’s permission to attempt to obtain the geolocation information must be sought before proceeding. The browser will take care of this, and a message will either appear as a popup box, or at the top of the browser (implementation is browser specific) requesting the user’s permission.
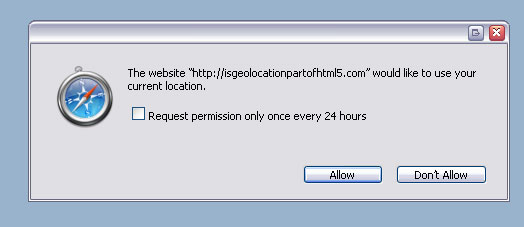
Geolocation sources
A number of different sources are used to attempt to obtain the user’s location, and each has their own varying degree of accuracy. A desktop browser is likely to use WiFi (accurate to 20m) or IP Geolocation which is only accurate to the city level and can provide false positives. Mobile devices tend to use triangulation techniques such as GPS (accurate to 10m and only works outside), WiFi and GSM/CDMA cell IDs (accurate to 1000m).
Using the API
Before you actually attempt to use the Geolocation API, you first need to check if the browser actually supports it. The API usefully provides a function for this which can be called as follows:
if (navigator.geolocation) {
// do fancy stuff
}
Obviously if this returns false, you should optionally inform the user of the inferiority of their browser and laugh in their face (not really).
Through the API, there are two functions available to obtain a user’s location:
getCurrentPosition and watchPosition
Both of these methods return immediately, and then asynchronously attempt to obtain the current location. They take the same number of arguments, two of which are optional:
successCallback
– called if the method returns successfully[errorCallback]
– called if the method returns with an error- [options] – a number of options are available:
enableHighAccuracy
– provides a hint that the application would like the best possible results. This may cause a slower response time and in the case of a mobile device, greater power consumption as it may use GPS. Boolean with a default setting of false.timeout
– indicates the maximum length of time to wait for a response. In milliseconds with a default of 0 – infinite.maximumAge
– denotes the maximum age of a cached position that the application will be willing to accept. In milliseconds, with a default value of 0, which means that an attempt must be made to obtain a new position object immediately.
Before mentioning the differences between the two, another method needs to be introduced:
clearWatch
This method takes one argument, the
watchID
of the watch process to clear (which is returned by watchPosition
)
Now, the main difference between
getCurrentPosition
andwatchPosition
is that watchPosition
keeps informing your code should the position change, so basically it keeps updating the user’s position. This is very useful if they’re on the move and you want to keep track of their position, whereas getCurrentPosition
is a once off. This method also returns a watchID
which is required when you want to stop the position constantly being updated by calling clearWatch
method is called.
When the user’s position is returned, it is contained within a
Position
object which contains a number of properties:Property | Details |
---|---|
coords.latitude | Decimal degrees of the latitude |
coords.longitude | Decimal degress of the longitude |
coords.altitude | Height in metres of the position above thereference ellipsoid |
coords.accuracy | The accuracy in metres of the returned result. The value of this setting informs the application how useful the returned latitude/longitude value actually is. This can help in determining if the returned result is accurate enough for the purpose it is intended for, e.g. values for streetview locations will need to be more accurate than those for a country based location |
coords.altitudeAccuracy | The accuracy in metres of the returned altitude |
coords.heading | Direction of travel of the hosting device, clockwise from true north |
coords.speed | The current ground speed of the hosting device in metres per second |
timestamp | Timestamp of when the position was acquired |
Amongst these only which is
coords.latitude
, coords.longitude
and coords.accuracy
are guaranteed to be returned (all others may benull
), and the first two are by and large the most relevant, as it is from these that a position can be plotted on a Google Map, for example.
You can read more about the
Position
object and the coordinates interfaceon the W3 specification itself.Putting it all together
Putting this altogether, the following code will attempt to obtain a user’s location, calling the method
displayPosition
on success which simply pops up an alert box with the captured latitude and longitude:if (navigator.geolocation) {
var timeoutVal = 10 * 1000 * 1000;
navigator.geolocation.getCurrentPosition(
displayPosition,
displayError,
{ enableHighAccuracy: true, timeout: timeoutVal,maximumAge: 0 }
);
}
else {
alert("Geolocation is not supported by this browser");
}
function displayPosition(position) {
alert("Latitude: " + position.coords.latitude + ", Longitude: " + position.coords.longitude);
}
The code above also calls the
displayError
method when attempt to fetch the user’s location data. This function simple converts the returned error code to an appropriate message:function displayError(error) {
var errors = {
1: 'Permission denied',
2: 'Position unavailable',
3: 'Request timeout'
};
alert("Error: " + errors[error.code]);
}
A final word
This is just a simple introduction to using the Geolocation API and one of it’s uses.
Of course the API can be used for more than simply plotting a position on a map (although that in itself is quite useful). The specification itself offers a list of potential use cases for the API such as:
- Finding and plotting points of interest in the user’s area
- Annotating content with location informationShowing a user’s position on a map (helping with directions of course!)
- Turn-by-turn route navigation – using
watchPosition
- Up-to-date local information – updates as you move
Examples
To see geolocation in action, I’ve put together a couple of quick examples in which I encourage you to view source and then go and try it out for yourself.
- Plot a location on a Google Map using
getPosition
- Plot and update a location on a Google Map using
watchPosition
– this is best viewed on a moving device so you can see the position updating